Widgets
Widgets are the components that make up a GTK application. GTK offers many widgets and if those don't fit, you can even create custom ones. There are, for example, display widgets, buttons, containers and windows. One kind of widget might be able to contain other widgets, it might present information and it might react to interaction.
The Widget Gallery is useful to find out which widget fits your needs.
Let's say we want to add a button to our app.
We have quite a bit of choice here, but let's take the simplest one — a Button
.
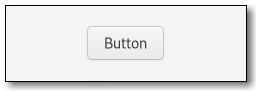
GTK is an object-oriented framework, so all widgets are part of an inheritance tree with GObject
at the top.
The inheritance tree of a Button
looks like this:
GObject
╰── Widget
╰── Button
The GTK documentation also tells us that Button
implements the interfaces GtkAccessible
, GtkActionable
, GtkBuildable
, GtkConstraintTarget
.
Now let's compare that with the corresponding Button
struct in gtk-rs
.
The gtk-rs documentation tells us which traits it implements.
We find that these traits either have a corresponding base class or interface in the GTK docs.
In the "Hello World" app we wanted to react to a button click.
This behavior is specific to a button, so we expect to find a suitable method in the ButtonExt
trait.
And indeed, ButtonExt
includes the method connect_clicked
.
Filename: listings/hello_world/3/main.rs
use gtk::prelude::*;
use gtk::{glib, Application, ApplicationWindow, Button};
const APP_ID: &str = "org.gtk_rs.HelloWorld3";
fn main() -> glib::ExitCode {
// Create a new application
let app = Application::builder().application_id(APP_ID).build();
// Connect to "activate" signal of `app`
app.connect_activate(build_ui);
// Run the application
app.run()
}
fn build_ui(app: &Application) {
// Create a button with label and margins
let button = Button::builder()
.label("Press me!")
.margin_top(12)
.margin_bottom(12)
.margin_start(12)
.margin_end(12)
.build();
// Connect to "clicked" signal of `button`
button.connect_clicked(|button| {
// Set the label to "Hello World!" after the button has been clicked on
button.set_label("Hello World!");
});
// Create a window
let window = ApplicationWindow::builder()
.application(app)
.title("My GTK App")
.child(&button)
.build();
// Present window
window.present();
}